[Techniczne] Nasłuchiwanie wydarzeń na stronie www
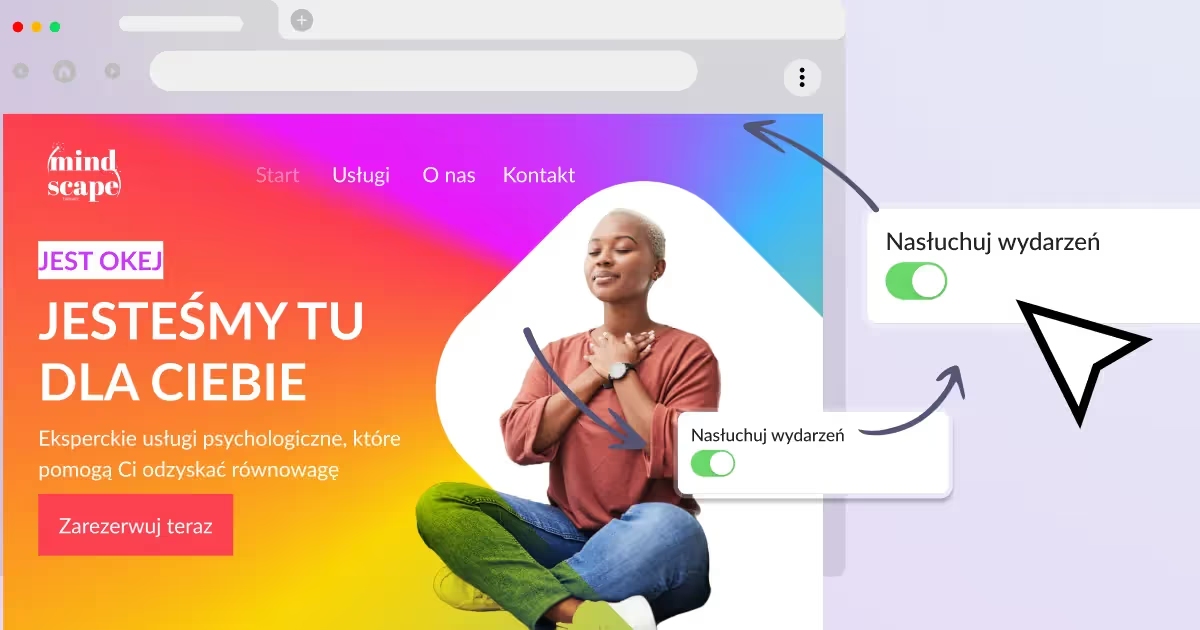
![[Techniczne] Nasłuchiwanie wydarzeń na stronie www](https://cdn.prod.website-files.com/65a722edc8c9641642f1ab91/65e163516ab08d791fa88aea_placeholder_3.webp)
Ten artykuł jest artykułem technicznym i wymaga wiedzy programistycznej (podstaw znajomości JavaScript).
System rezerwacyjny Calendesk może wysyłać tzw. eventy po wystąpieniu określonych wydarzeń, które mają miejsce na stronie www utworzonej przez system Calendesk.
Możesz reagować na te wydarzenia, wykorzystując własny kod, który umieścisz w funkcji, która będzie wywoływana przez Calendesk.
Ta opcja przyda się, kiedy np. chcesz mierzyć konwersję na stronie www do określonych akcji, chcesz wyświetlić dodatkowe informacje klientowi lub w innych niestandardowych przypadkach.
Lista globalnych funkcji, które będą wywoływane po wystąpieniu wydarzeń:
- Utworzenie konta klienta
calendeskUserCreated
- Utworzenie rezerwacji
calendeskBookingsCreated
- Opłacenie rezerwacji – sukces
calendeskBookingPaymentSuccessful
- Opłacenie rezerwacji – błąd
calendeskBookingPaymentUnsuccessful
- Zmiana terminu rezerwacji
calendeskBookingsRescheduled
- Anulowanie rezerwacji
calendeskBookingsCanceled
- Płatność za rezerwację za pomocą subskrypcji
calendeskUserSubscriptionPaidForBooking
- Utworzenie subskrypcji
calendeskUserSubscriptionCreated
- Anulowanie subskrypcji
calendeskUserSubscriptionCanceled
Aby reagować na powyższe wydarzenia, wystarczy, że zadeklarujesz funkcję globalną w swoim kodzie, zgodną z powyższymi nazwami i wstawisz ją na stronę w Calendesk.
Przykładowe funkcje, które możesz wykorzystać w swojej implementacji, wraz z opisem:
1async function calendeskUserCreated(user) {
2 // We will call this function after successful user signup. A parameter `user` is a user object.
3 // User object:
4 // public id!: number
5 // public name!: string | null
6 // public surname!: string | null
7 // public email?: string | null
8 // public cardBrand?: string | null
9 // public cardLastFour?: string | null
10 // public stripeId?: string | null
11 // public status!: UserStatus
12 // public preferences?: UserPreferences | null
13 // public subscriptions?: Array<UserSubscription>
14 // public defaultImage!: DefaultImage
15 // public defaultAddress?: Address | null
16 // public defaultPhone?: Phone | null
17 // public dateOfBirth?: string | null
18 // public emailVerifiedAt?: string | null
19
20 console.log('Function: calendeskUserCreated, User: ', user)
21}
22
23async function calendeskBookingsCreated(bookings) {
24 // We will call this function after creating a booking. A parameter `bookings` is an array of booking objects.
25 // Booking object:
26 // public id!: number
27 // public employeeId!: number
28 // public serviceId!: number
29 // public serviceTypeId!: string
30 // public startDate!: string
31 // public endDate!: string
32 // public startTime!: string
33 // public endTime!: string
34 // public startsAt!: string
35 // public endsAt!: string
36 // public customerTimeZone!: string
37 // public status!: BookingStatus
38 // public control!: string
39 // public location!: ServiceLocation
40 // public paid!: boolean
41 // public paymentMethod!: string
42 // public paidWithUserSubscriptionId!: string | null
43 // public googleMeetUrl!: string
44 // public zoomJoinUrl!: string
45 // public teamsUrl!: string
46 // public skypeUrl!: string
47 // public customerWhatsAppUrl!: string
48 // public employeeWhatsAppUrl!: string
49 // public customFields!: Array<CustomField> | null
50 // public paymentTransaction!: string
51 // public service!: Service
52 // public serviceType!: ServiceType
53 // public employee!: Employee
54 // public getPrice (): number;
55 // public canBeCanceled (): boolean;
56 // public getDuration (): number;
57
58 console.log('Function: calendeskBookingsCreated, Bookings: ', bookings)
59}
60
61async function calendeskBookingPaymentSuccessful(booking) {
62 // We will call this function after successful booking payment. A parameter `booking` is a booking object.
63 console.log('Function: calendeskBookingPaymentSuccessful, Booking: ', booking)
64}
65
66async function calendeskBookingPaymentUnsuccessful(booking) {
67 // We will call this function after unsuccessful booking payment. A parameter `booking` is a booking object.
68 console.log('Function: calendeskBookingPaymentUnsuccessful, Booking: ', booking)
69}
70
71async function calendeskBookingsRescheduled(booking) {
72 // We will call this function after successful booking reschedule. A parameter `booking` is a booking object.
73 console.log('Function: calendeskBookingsRescheduled, Booking: ', booking)
74}
75
76async function calendeskBookingsCanceled(booking) {
77 // We will call this function after successful booking cancelation. A parameter `booking` is a booking object.
78 console.log('Function: calendeskBookingsCanceled, Booking: ', booking)
79}
80
81async function calendeskUserSubscriptionPaidForBooking(bookingIds) {
82 // We will call this function after paying with a subscription for a booking. A parameter `bookingIds` is an array of paid booking ids.
83 console.log('Function: calendeskUserSubscriptionPaidForBooking, Booking Ids: ', bookingIds)
84}
85
86async function calendeskUserSubscriptionCreated(subscription, user) {
87 // We will call this function after creating a subscription.
88 // A parameter `subscription` is a subscription object, a parameter `user` is a user object.
89 // Subscription object
90 // public id!: number
91 // public subscriptionId!: string
92 // public name!: string
93 // public description!: string | null
94 // public successUrl!: string | null
95 // public recurringInterval!: string | null
96 // public intervalCount!: number | null
97 // public serviceLimit!: number | null
98 // public wantsInvoice!: boolean
99 // public requireBillingData!: boolean
100 // public disableRobotIndexing!: boolean
101 // public tax!: string | null
102 // public createdAt!: string
103 // public updatedAt!: string
104 // public price!: SubscriptionProductPrice
105 // public services!: Array<Service> | null
106
107 // After calling this method, the system can redirect your user to the payment provider.
108 // To make sure that your data is sent correctly, please use the `await` option.
109 // For example, await new Promise(r => setTimeout(r, 2000))
110 console.log('Function: calendeskUserSubscriptionCreated, Subscription: ', subscription, 'User: ', user)
111}
112
113async function calendeskUserSubscriptionCanceled(userSubscription, user) {
114 // We will call this function after successful subscription cancelation.
115 // A parameter `userSubscription` is a user subscription object, a parameter `user` is a user object.
116 // User Subscription object:
117 // public id!: number
118 // public subscriptionId!: number
119 // public stripeSubscriptionId!: number
120 // public canceledAt!: string | null
121 // public endsAt!: string | null
122 // public createdAt!: string
123 // public status!: SubscriptionStatus
124 // public subscription!: Subscription
125 // public usageRecords!: Array<UserSubscriptionUsageRecord> | null
126 // public isActive (): boolean
127
128 console.log('Function: calendeskUserSubscriptionCanceled, User subscription: ', userSubscription, 'User: ', user)
129}
Gdzie wkleić funkcje?
Funkcje możesz wkleić w kreatorze stron w okienku Skrypty niestandardowe, pomiędzy tagi script, np.
1<script>
2async function calendeskBookingsCreated(bookings) {
3 // Your code that will be executed after creating a booking.
4 // For example, if you use Google Analytics, you can call here a conversion script for bookings.
5}
6</script>
Zobacz powiązany artykuł Jak podłączyć Google Analytics do strony zrobionej w Calendesk?

Przydatne przykłady
Wyślij zdarzenie konwersji do Google Tag Manager za pomocą Data Layer:
1<script>
2function calendeskBookingsCreated(bookings) {
3 window.dataLayer = window.dataLayer || [];
4 for (var i = 0; i < bookings.length; i++) {
5 var booking = bookings[i]
6 dataLayer.push({
7 'event': 'bookingCreated',
8 'bookingId': booking.id,
9 'employeeId': booking.employeeId,
10 'serviceId': booking.serviceId,
11 'serviceTypeId': booking.serviceTypeId,
12 'startDate': booking.startDate,
13 'endDate': booking.endDate,
14 'startTime': booking.startTime,
15 'endTime': booking.endTime,
16 'startsAtUTC': booking.startsAt,
17 'endsAtUTC': booking.endsAt,
18 'customerTimeZone': booking.customerTimeZone,
19 'price': (booking.getPrice() / 100) // Convert cents to decimal
20 })
21 }
22}
23</script>
Wyślij zdarzenie konwersji do Google Analytics:
1<script>
2function calendeskBookingsCreated(bookings) {
3 if (typeof ga === 'undefined') {
4 console.error('Google Analytics not loaded');
5 return;
6 }
7 // Loop through the bookings array and send each booking as an event to GA
8 for (var i = 0; i < bookings.length; i++) {
9 var booking = bookings[i];
10 // Convert price from cents to decimals
11 var priceInDecimals = booking.getPrice() / 100;
12 // Convert price to an integer if it's a float, e.g. by rounding
13 var priceAsInteger = Math.round(priceInDecimals);
14 ga('send', 'event', {
15 eventCategory: 'booking',
16 eventAction: 'bookingCreated',
17 eventLabel: `Booking ID: ${booking.id}, Employee ID: ${booking.employeeId}, Service ID: ${booking.serviceId}, Service Type ID: ${booking.serviceTypeId}`,
18 eventValue: priceAsInteger,
19 nonInteraction: true
20 });
21 }
22}
23</script>